Introduction to Node.js
Introducing a truly understandable Node.js tutorial series for beginner and intermediate developers.
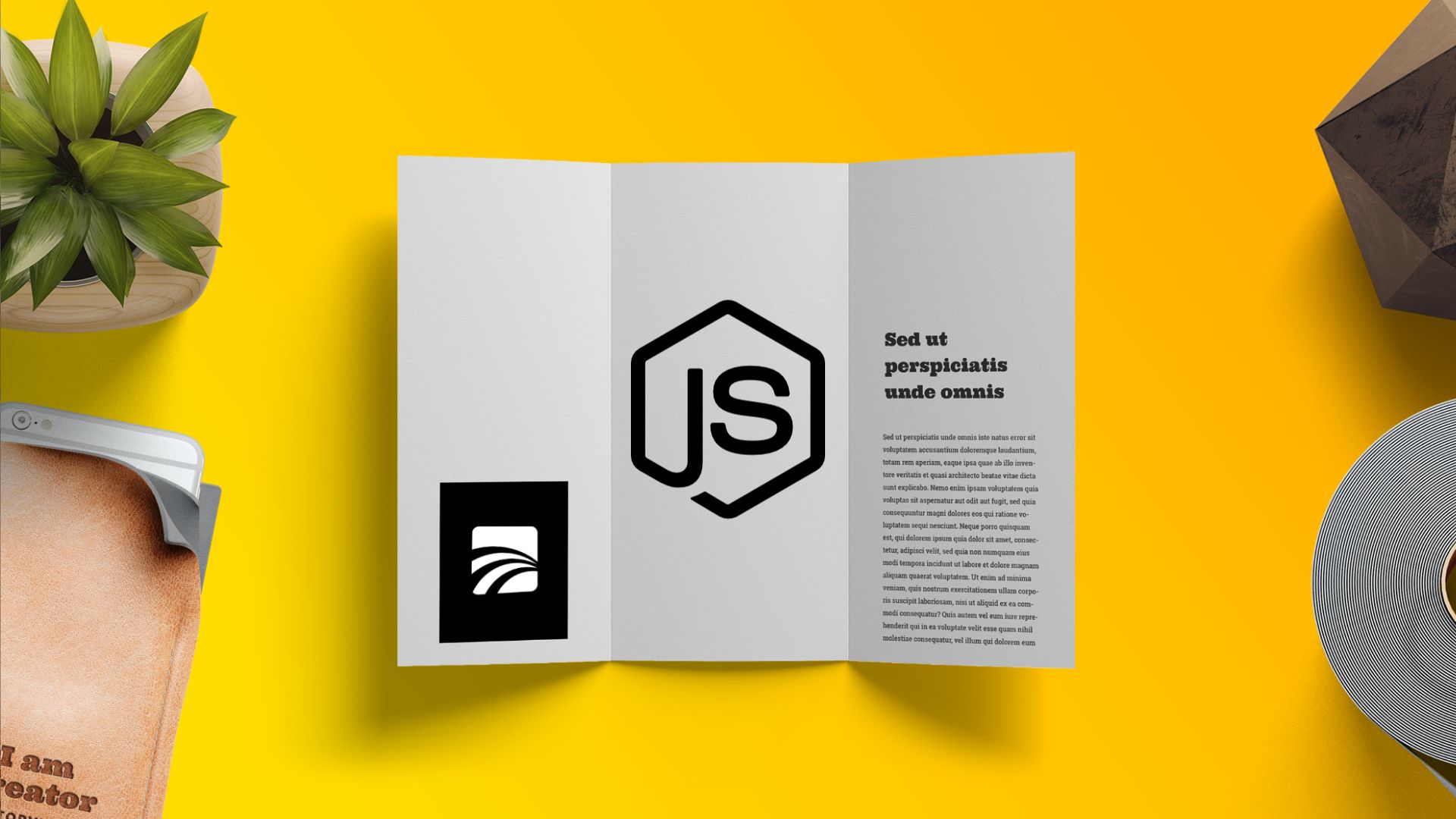
Introducing a truly understandable Node.js tutorial series for beginner and intermediate developers.
Whether you're just starting out or you already have some Node.js experience, this tutorial will guide you through all you need to know to find your way trough any existing Node.js project, as well as writing your own Node.js projects from scratch. Except for having some experience with Javascript, there's no prior knowledge needed.
I'm writing this tutorial series because when I started out, everything about Node.js seemed so complicated. The code sample on the official website made it seem like it's some complicated server language, and I didn't know how it compares to what I previously knew. Having come from PHP and Ruby, it felt like I wouldn't be able to write the same things using Javascript. Fortunately, nothing could be further from the truth.
In this tutorial you'll learn what Node.js is, what it isn't, how it's going to help your development career and how you can use it to be more productive in your web development projects. Let's start!
What is Node.js?
Node.js is V8, the JavaScript engine running inside Google Chrome, bundled together with a couple of libraries for I/O (Input/Output) operations. Basically, it's what Chrome is using to interact with files on your computer using code written in Javascript.
Where does all the confusion come from? Node.js isn't any special variation of Javascript, it is just plain, modern Javascript, but it's running everywhere instead of just your browser.
When running Javascript in the browser, you're enclosed in a sandbox environment that doesn't allow you to interact with files on your computer, ensuring a secure browsing environment for the site's visitors, while executing Javascript using Node.js is meant to behave like a standard programming language.
Node.js became so popular because it allows you to use Javascript on both front-end and back-end. This way, every developer in your team can understand what’s happening on the entire stack, and make changes if necessary.
Why should you learn Node.js?
The two big mainstream uses are web application servers and asset pipeline automation.
Node.js uses an event-driven, non-blocking input/output model. Node.js scales flawlessly, and works perfectly for high-throughput server-side applications. Translated into simple English, this means that Node.js can be used to write applications that can handle large numbers of concurrent requests.
However, Node.js may not provide everything you need from a language out of the box. Many times you'll find yourself needing additional functionality, that you'll usually find available as a nicely maintained open source package. If you enjoy building things out of reusable blocks, then Node.js is definitely the for you. I sure do.
Node.js Goodies
More reasons to learn Node.js are Express, npm, and Webpack.
Express is a modular application server for Node.js that allows you to write scalable web applications. Express focuses on code reusability and development costs, giving you a strong foundation for your application. It's lightweight and super fast.
Node's package manager "npm" is a very powerful tool that can be used to share your code as packages. Not only that, but it allows you to reuse code written by other developers inside your own package, as dependencies. Just imagine having more than 600,000 packages at your fingertips.
Surely, everyone developer has heard at least once about Webpack by now. Webpack is a build tool that puts all of your assets, including CSS, Javascript, images, and even fonts, in a dependency graph. Webpack lets you use require()
in your source code to point to local files, and decide how they're processed in your final bundle. If you're building a complex front-end application with many static assets that require processing, then Webpack will give you great benefits.
Still not convinced?
Node.js and Javascript have been voted as the most popular development framework and programming in the 2018 StackOverflow Developer Survey.
For the sixth year in a row, Javascript is the most commonly used programming language. It's amazing how popular the Node.js stack has become and you shouldn't be missing out!
What you already need to know
As you might have thought, there are some prerequisites before you can start learnng Node.js. When I started out, I had a strong programming and web development background, so the learning curve was almost non-existent.
-
Javascript ― Node.js is basically server side Javascript, so knowing Javascript is an absolute must. If you're familiar with other programming languages, learning Javascript will be fairly straightforward.
-
HTTP ― If you're building web applications, you will need to understand HTTP client and server paradigm. You'll do just fine with some basic understanding and knowing what verbs like GET, POST, PUT, and DELETE are used for.
-
Asynchronous Programming ― JavaScript is asynchronous in nature and so is Node.js. For example, Node.js will not wait for a HTTP request's response to arrive before executing the next line of code, and you'll rely heavily on callback functions.
Besides these, I'd recommend trying to understand what the concept of REST is and how it works, and reading up about the Node.js Event Loop. It might all seem like a lot to learn, but in reality they're simple concepts with fancy names.
What's next?
You'll learn how to create our own package and add dependencies to it later in this tutorial. Next, head over to Installing Node.js the Right Way, to install Node.js, write and run your first Node.js "Hello world!" program.